This project (see ./examples/logaxes/
) simply creates a JKQTPlotter widget (as a new window) and several line-graphs of different resonance curves.
The source code of the main application can be found in logaxes.cpp
. Mainly several graphs are generated in a loop and then different line styles are applied to the graphs (set by `graph->setLineStyle()
). The colors are set automtically from an internal default palette. The main loop looks like this:
QVector<Qt::PenStyle> pens {Qt::SolidLine, Qt::DashLine, Qt::DotLine, Qt::DashDotLine, Qt::DashDotDotLine };
for (int id=0; id<D.size(); id++) {
QVector<double> Y;
for (auto& xx: X) {
Y<<1.0/sqrt(sqr(1-sqr(xx))+sqr(2*xx*D[id]));
}
graph->
setYColumn(ds->addCopiedColumn(Y,
"y"+QString::number(
id)));
graph->
setTitle(QString(
"D=\\delta/\\omega_0=%1").arg(D[
id]));
plot.addGraph(graph);
}
void setLineWidth(double __value)
set the line width of the graph line (in pt)
void setLineStyle(Qt::PenStyle __value)
set the style of the graph line
void setSymbolType(JKQTPGraphSymbols __value)
set the type of the graph symbol
virtual void setTitle(const QString &__value)
sets the title of the plot (for display in key!).
void setYColumn(int __value)
the column that contains the y-component of the datapoints
void setXColumn(int __value)
the column that contains the x-component of the datapoints
This implements xy line plots. This also alows to draw symbols at the data points.
Definition jkqtplines.h:61
Then a JKQTPGeoText
is added to the graph, which shows the function plotted in the plot:
plot.addGraph(
new JKQTPGeoText(&plot, 1.25, 10,
"$\\frac{A}{A_{stat}}=\\frac{1}{\\sqrt{\\left(1-\\eta^2\\right)^2+\\left(2{\\eta}D\\right)^2}}$", 15, QColor(
"black")));
This JKQTPPlotAnnotationElement is used to display text. It uses the JKQTMathText class in order to d...
Definition jkqtpgeoannotations.h:185
The difference between not using and using $...$
for the equation can be seen here:
- no $-math-mode:
- using $-math-mode:
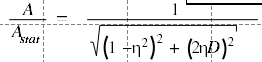
Finally the y-axis is switched to logarithmic scaling and the axis labels are set:
plot.getYAxis()->setLogAxis(true);
plot.getYAxis()->setMinorTicks(9);
plot.getYAxis()->setDrawMinorGrid(true);
plot.getYAxis()->setLabelDigits(0);
plot.getYAxis()->setTickLabelFontSize(10);
plot.getYAxis()->setMinorTickLabelFontSize(7);
plot.getYAxis()->setLabelFontSize(14);
plot.getXAxis()->setTickLabelFontSize(10);
plot.getXAxis()->setMinorTickLabelFontSize(7);
plot.getXAxis()->setLabelFontSize(14);
plot.getYAxis()->setAxisLabel("Amplitude $A/A_{stat}$");
plot.getXAxis()->setAxisLabel("relative driving frequency $\\eta=\\omega/\\omega_0$");
@ JKQTPCALTexponent
show numbers in exponential for, e.g. ...
Definition jkqtptools.h:453
As an alternative JKQTPCALTexponentCharacter
does not use the power-of-10 notation, but uses the usual unit-characters, e.g. 0.001=1m, 0.000001=1ยต, 10000=10k, ...
The result looks like this:
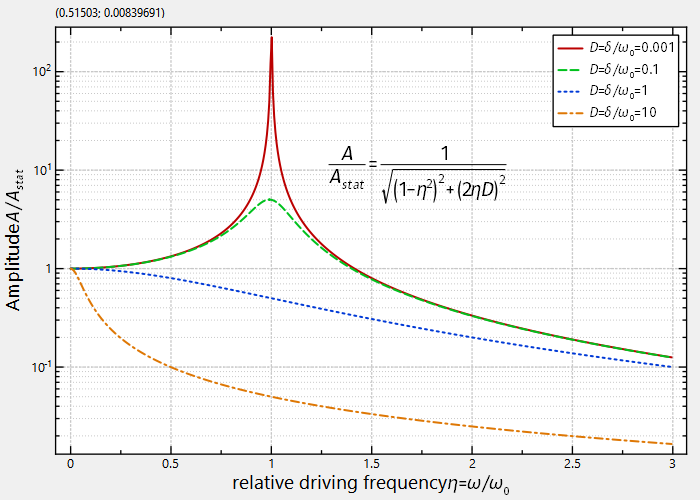
Without the logarithmic scaling we would have:
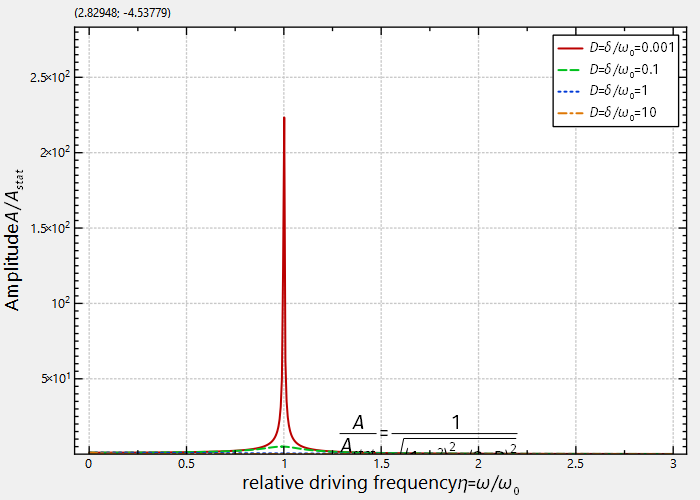
Switching the minor grid off results in a plot like this:
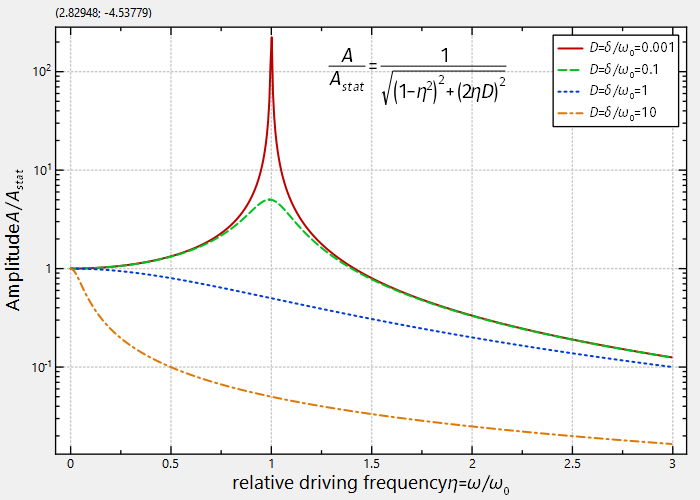
These examples show the results for different typical values for setMinorTicks()
:
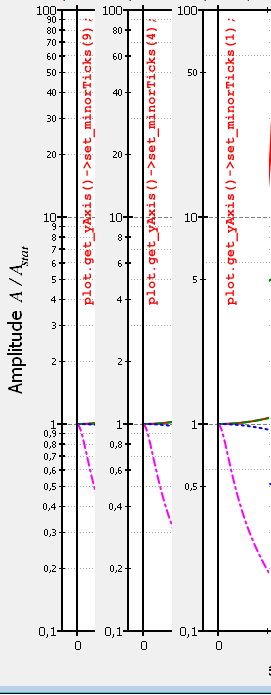
These examples show the results for different typical values for setLabelType()
: